Table of contents
Pencil Data Types
Pencil supports various data types for shape properties. Some of them are equivalent to data types in popular programming languages while the others are for convenience.
This document lists all supported data types in Pencil, each with both JavaScript syntax and XML syntax for use in stencil coding. Most of the supported data types have at least one way end-user can modify the value.
Alignment
Data structure for storing two-dimension alignment.
Construction:new Alignment(h, v);XML syntax:
<Property name="test" displayName="Test" type="Alignment">h,v</Property>
- h (number): horizotal alignment. 0: left, 1: center, 2: right
- v (number): vertical alignment. 0: top, 1: middle, 2: bottom
Members:
Name | Return Type | Description |
---|---|---|
static fromString(String) | Alignment | Builds the Alignment object from its string presentation.var align = Alignment.fromString("10,10"); |
toString() | String |
var s = align.toString(); // return "10,10" |
Editor supports:
Property page: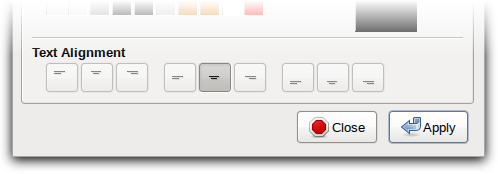
Properties of type Alignment
can be edited in the
shape property page.
Bool
Data type for storing boolean values.
Construction:new Bool(value);XML syntax:
<Property name="sample" displayName="Sample" type="Bool">value</Property>where value is either true or false
Members:
Name | Return Type | Description |
---|---|---|
static fromString(String) | Bool | Builds the Bool object from its string presentation.var b = Bool.fromString("true"); |
toString() | String | Return the string presentation of the boolean value. |
negative() | Bool | Return the Bool object with negative value. |
Editor supports:
Context menu: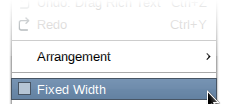
Properties of type Bool
can be edited in the
context menu of the shape using a checkbox item.
Dimension
Data structure for storing object size, a pair of width and height values
Construction:new Dimension(width, height);XML syntax:
<Property name="box" displayName="Box" type="Dimension" p:lockRatio="true">width,height</Property>
Members:
Name | Return Type | Description |
---|---|---|
static fromString(String) | Dimension | Build a Dimension object from its string presentation.var box = Dimension.fromString("10,10"); |
toString() | String |
var s = box.toString(); // return "10,10" |
narrowed(paddingX[, paddingY]) | Dimension | Return a new Dimension object with is created by narrowing the
callee by the provided paddings. If paddingY is omitted, paddingX
will be used for both directions.var box1 = box.narrowed(5); var box2 = box.narrowed(5, 10); |
p:lockRatio | Meta constraint used in XML syntax to hint that the ratio of
this object need to be maintained when its width and height are
changed. |
Editor supports:
On-canvas editor: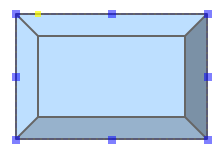
A Dimension
property with the special name of
box
can be edited using the on-canvas
geometry editor.

And also via the geometry toolbar located on the top of the Pencil application window.
Bound
Data structure for storing a bounding box which is a composite of a location and a size.
Construction:new Bound(left, top, width, height);Example:
var testBound = new Bound(10, 10, 10, 10);
Name | Return Type | Description |
---|---|---|
static fromBox(box, paddingX, paddingY) | Bound | Generating a new Bound object from a Dimension object narrowed
down on each sides using the provided paddingsvar b = Bound.fromBox(box, x, y); //equals to: var b = new Bound(x, y, box.w - 2 * x, box.h - 2 * y) |
static fromString(String) | Bound | Build a Bound object from its string presentationvar bound = Bound.fromString("10, 10, 10, 10"); |
toString() | String | Returns the string presentation of the callee. |
narrowed(paddingX, paddingY) | Bound | Create a new Bound object by using the callee and narrowing
down each sides by the provided paddingsvar bound2 = bound.narrowed(5, 8); |
Color
Data structure for storing object color with alpha blending
Construction:var color = new Color(); // create an opaque black colorXML syntax:
<Property name="color" displayName="My Color" type="Color">#000000ff</Property>
Name | Return Type | Description |
---|---|---|
static fromString(String) | Color | Build a color object from string presentation in CSS numerical
color syntax.Examples: Color.fromString("#ffffffff"); // solid white Color.fromString("#ffffff"); // also solid white Color.fromString("rgb(255, 0, 0)"); // solid red // semi-transparent blue: Color.fromString("rgba(0, 0, 255, 0.5)"); Color.fromString("transparent"); //transparent //semi-transparent black: Color.fromString("#00000033"); |
toString() | String | Returns the extended hexa string presentation of the color: #RRGGBBAA |
toRGBString() | String | Return the CSS color in the format of "rgb(red, green,
blue)"var color = Color.fromString("#FFFFFFFF"); color.toRGBString(); // rgb(255, 255, 255) |
toRGBAString() | String | Return the CSS color in the format of "rgba(red, green, blue,
alpha)"var color = Color.fromString("#FFFF0077"); color.toRGBAString(); // rgba(255, 255, 0, 0.5) |
shaded(percent) | Color | Creates a darker version of a color using the provided percent.
color.shaded(0.1); // returns a 10% darker color |
hollowed(percent) | Color | Creates a more transparent version of a color by the provided
percent.
color.hollowed(0.1); // returns a 10% more transparent color |
inverse() | Color | Returns the inversed version of a color |
transparent() | Color | Creates a fully transparent version of a color |
Editor supports:
Property page: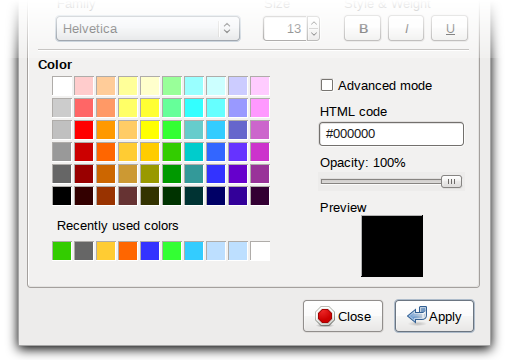
Properties of type Color
can be edited in the
property dialog with a color chooser that supports both simple and
advanced mode.
Color
properties with the following special names
can be also edited with the Color toolbar: textColor
,
fillColor
and strokeColor
.
CSS
Provides a data object for styling SVG elements and HTML elements.
Construction:var css = new CSS();
Name | Return Type | Description |
---|---|---|
set(name, value) | CSS | Sets a CSS property value, overriding existing one if any and
returns the object itself.css.set("font-size", "12px"); |
toString() | String | Return a string containing all specified properties.var css = new CSS(); css.set("font-size", "12px"); css.set("font-weight", "bold"); var s = css.toString(); // returns "font-size: 12px; font-weight: bold;" |
clear() | CSS | Removes all properties contrained in a CSS object and returns the object itself. |
unset(name) | CSS | Removes a specific property from a CSS object if any and returns the object itself. |
get(name) | String | Get the property’s value. |
contains(name) | Bool | Check whether a CSS object contains the property.
css.contains("font-size"); |
setIfNot(name, value) | CSS | Sets value to a property if it was not set, returns the object
itself Ex:css.setIfNot("font-size", "12px") |
static fromString(literal) | CSS | Parses the CSS string and creates a CSS object containing all
parsed property/value pairs.CSS.fromString("font-size: 12px; font-weight: bold"); |
importRaw(literal) | void |
Parses the CSS string and add all parsed property/value pairs
to the calle overriding any existing properties.var css = new CSS(); css.set("color", "#FF0000"); css.importRaw("font-size: 12px; font-weight: bold"); |
Enum
Data structure to store option with the possibility to specify available options via XML metadata.
XML syntax:<Property name="type" displayName="Type" type="Enum" p:enumValues="['one|One', 'two|Two']">two</Property>
Name | Return Type | Description |
---|---|---|
value | String | Member field storing the selected value. |
p:enumValues | String | An array literal containing all possible options. Each option
is in the syntax of 'id|Display Name' .
... p:enumValues="['linux|GNU Linux', 'win32|Win 32']" |
Editor supports:
Context menu: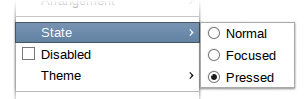
Properties of type Enum
can be edited in the
context menu of the shape.
Font
Data structure for manipulating font info.
Construction:var font = new Font();XML syntaxt:
<Property name="textFont" displayName="Text Font" type="Font">{families}|{weight}|{style}|{size}[|{decor}]</Property>
Name | Return Type | Description |
---|---|---|
static fromString(String) | Font | Build a Font object from its string presentation.var f = Font.fromString("Arial|bold|italic|12px"); |
getPixelHeight() | Number | Return the font height in pixels;
var f = Font.fromString("Arial|bold|italic|12px"); var height = f.getPixelHeight(); // 12 |
toString() | String | Return a string representing the font object. |
toCSSFontString() | String | Return the string presentation of the font object in CSS
syntax.var f = Font.fromString("Arial|bold|italic|12px"); var css = f.toCSSFontString(); // "Arial bold italic 12px" |
getFamilies() | String | Returns the families field of the font. |
Editor supports:
Property page: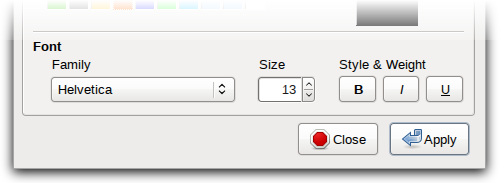
Properties of type Font
can be edited in the
property dialog.
Font
property with the special name
textFont
is editable with the Font style toolbar.
Handle
Provides a special data object representing a point in 2D coordinate and can be modified on the drawing canvas by user operations.
Construction:<Property name="a" displayName="Start Point" type="Handle">x,y</Property>
Name | Input value | Description |
---|---|---|
x | number | Distance to the left border of the shape |
y | number | Distance to the top border of the shape |
p:lockX | boolean expr. | The 'x' value should not be changed, horizontal movement is
disabled. Default value: false
Example: .... p:lockX="$fixedWidth.value == true" |
p:lockY | boolean expr. | The 'y' value should not be changed, vertical movement is
disabled. Default value: false
Example: .... p:lockY="$fixedHeight.value == true" |
p:minX | number expr. | Minimum value of 'x'. Movement of the handle should not pass
this lower limit.Example: .... p:minX="15" |
p:maxX | number expr. | Maximum value of 'x'. Movement of the handle should not pass
this upper limit.Example: .... p:maxX="$box.w / 2" |
p:minY | number expr. | Minimum value of 't'. Movement of the handle should not pass
this lower limit.Example: .... p:minY="$box.h / 3 + 10" |
p:maxY | number expr. | Maximum value of 'y'. Movement of the handle should not pass
this upper limit.Example: .... p:minY="$box.h - 10" |
p:noScale | true | false | Disable auto-scaling of Handle value when the object 'box' property is changed. Default value: false |
Editor supports:
On-canvas editor: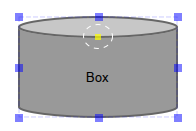
Each property of type Handle
is shown as a yellow
bullet when the shape is focused. The property can be edited by
moving that bullet.
ImageData
Data structure that store a binary bitmap image.
Construction:new ImageData(w, h, dataUrl);XML syntax:
<Property name="image" displayName="Image" type="ImageData"><![CDATA[w,h,url]]></Property>Example:
var image = new ImageData(10, 15, "data:image/png;base64,iVBORw0KQmCC...");
Name | Return Type | Description |
---|---|---|
w | number | The image width |
h | number | The image height |
data | string | The data url of the image. |
static fromString(String) | ImageData | Builds an ImageData object from its string presentation.var i = ImageData.fromString("57,57, data:image/png;base64,iVBORw0KQmCC"); |
toString() | String | Returns the string presentation of the callee. |
PlainText
Data object that represents a piece of plain texts.
Construction:var text = new PlainText("Pugnabant totidemque vos nam");XML syntax:
<Property name="text" displayName="Text" type="PlainText"><![CDATA[Pugnabant totidemque vos nam]]></Property>
Name | Return Type | Description |
---|---|---|
value | String | Data field containing the text. |
static fromString(String) | PlainText | Builds a PlainText object from the provided JS string |
toString() | String | Returns the contained text. |
toUpper() | PlainText | Returns a uppercase version of the callee. |
Editor supports:
On-canvas editor: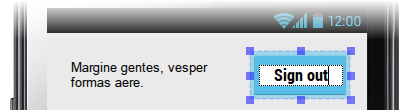
PlainText
properties can be edited right on the
canvas using a simple text input.
RichText
Data structure for storing rich-text content in HTML format.
Construction:var text = new RichText("A <b>rich</b> text string");XML syntax:
<Property name="text" displayName="Text" type="RichText"><![CDATA[A <b>rich</b> text string]]></Property>
Name | Return Type | Description |
---|---|---|
value | String | Data field containing the HTML text. |
static fromString(String) | RichText | Builds a RichText object from the provided JS string |
toString() | String | Returns the contained HTML text. |
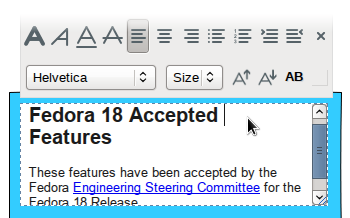
Editor supports:
On-canvas editor:RichText
properties can be edited right on the
canvas using a rich-text input.
StrokeStyle
Data structure storing stroke styling information.
Construction:new StrokeStyle(width, dasharray);
The dasharray
value is specified as a JavaScript
array containing lengths of dashes and spaces. More information can
be found in the SVG
Specification for Stroke dash array.
<Property name="stroke" type="StrokeStyle" displayName="Border Style">w|dasharray</Property>
When the dasharray
is omitted, the stroke is
considered solid.
var stroke = new StrokeStyle("1,[4,2,1,2]"); // construct a 'dash-space-dot-space' stroke at 1px widthOr in xml format:
<Property name="stroke" type="StrokeStyle" displayName="Border Style">1|[4,2,1,2]</Property>
Name | Return Type | Description |
---|---|---|
static fromString(String) | StrokeStyle | Build a StrokeStyle object from its string presentation.var ss = StrokeStyle.fromString("1|[1,1]"); |
toString() | String | Returns the string presentation of the callee. |
condensed(ratio) | StrokeStyle | Returns a new version of the callee by condensing the width by
the provided ration.
var stroke2 = stroke.condensed(-0.1); |
Editor supports:
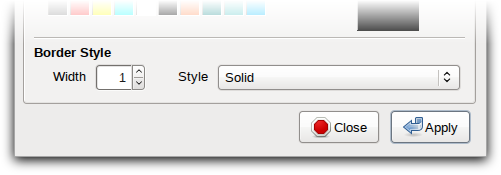
StrokeStyle
properties can be edited in the
property page of the shape.
ShadowStyle
Data structure that stores shadow style information.
Construction:var shadow = new ShadowStyle(dx, dy, size);XML syntax:
<Property name="shadow" type="ShadowStyle" displayName="Box Shadow">dx|dy|size</Property>
Name | Return Type | Description |
static fromString(String) | ShadowStyle | Builds a ShadowStyle object from its string presentation.
var style = ShadowStyle.fromString("3|3|10"); |
toString() | String | Returns the string presentation of the callee. |
toCSSString() | String | Return the string presentation of the callee in CSS syntax. |
Editor supports:

ShadowStyle
properties can be edited in the
property page of the shape.